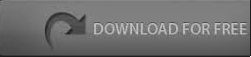
By now, you must have completed the implementation of the ADT and of a program that exercises that implementation (which we called adt-test.c in the pre-lab). Once you have that, the producer and consumer can safely call circular_list_insert and circular_list_removein a multi-threaded context because the synchronized ADT takes proper care of things. Now that you have a single-thread implementation for these functions, you can work to build synchronization into them. Int circular_list_remove(struct circular_list *l, item *i) * i pointer to an item onto which the removed item is copied Int circular_list_insert(struct circular_list *l, item i) * i item to copy into a position of the circular list Int circular_list_create(struct circular_list *l, int size) * 0 if successful, -1 if any error condition is found * size number of items to allocate in circular list * Create a circular list with a pre-defined buffer size. The work you did for the pre-lab implements the following application programming interface (API):
PRODUCER CONSUMER WITH BOUNDED BUFFER BINARY SEMAPHOR CODE
Primarily, there is an issue of applying the concept of abstraction: the code would be substantially easier to manage if you had an ADT for the circular-list. Looking at the structure of code given above, you will realize that having the producers and the consumer processes deal directly with synchronization is not ideal.
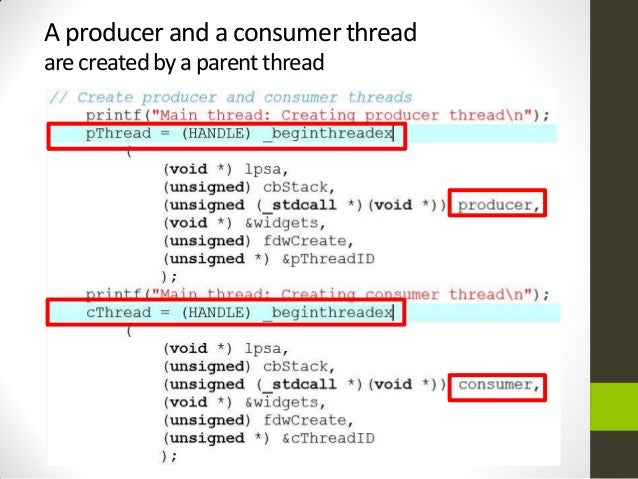
The solution to the problem is presented in structures that delineate the code for the two types of process as shows below. Your textbook, Operating Systems Concepts 10th Ed., by Silberschatz, Galvin, and Gagne, discusses the bounded-buffer problem in the context of the classical synchronization problem of producers and consumers (Section 7.1). By virtue of their scheduling, threads might interrupt each other in the middle of one of these operations leaving the data structure in an inconsistent state. If this list is to be shared by multiple threads, you need to be careful with how you implement functionality to remove and to return a buffer to the data structure. circular-queue) with n buffers, each capable of holding a single value of data type double. Additional students files associated with this lab, as well as any existing solutions can be provided upon request by e-mail to: perronebucknelleduĪssume that you have a circular-list (a.k.a. Permission to reuse this material in parts or in its entirety is granted provided that this credits note is not removed. In this lab, you will learn to work with these two mechanisms for thread synchronization as you implement a solution to the bounded-buffer problem. Fortunately, POSIX gives you the more general-purpose semaphore in the sem_t data data type. Learn to work with Unix and Pthread synchronization mechanisms.The Pthread library offers the pthread_mutex_t data type, which is much like a binary semaphore and therefore somewhat of limited utility in the solution of synchronization problems.Which also makes them have bounded waiting. (B) No starvation happen because there is alteration between $P$ and Consumer. (A) Deadlock cannot happen has both producer and consumer are operating on different semaphores (no hold and wait ) So, consumer consumes an item before producer produces the next item.
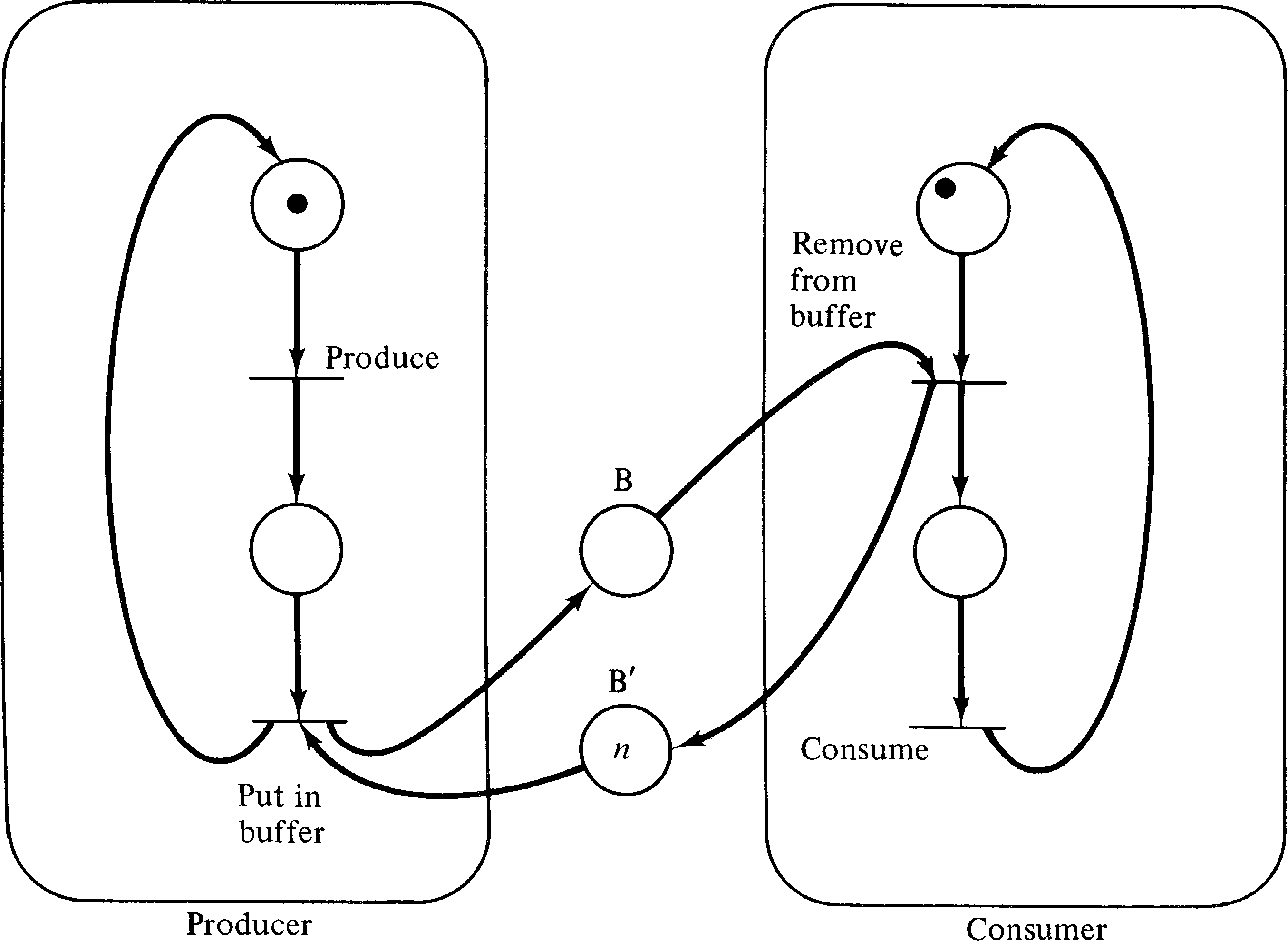
$P$ resumes from statement $2$, since it was blocked at statement $1$. value to $1$, wakes up the blocked process on Q 's queue.Hence process P is awaken. Up on $S$,now instead of changing the value of $S$. In the next iteration of while loop $st 1$ is executed.īut $S$ is already $0$, further down on $0$ sends $P$ to blocked list of $S.P$ is blocked.Įnters the statement $2$, consumes the item. This being an infinite while loop should infinitely iterate.
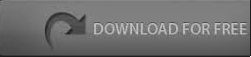